Welcome back to Salesforce Megha. Today, we’re diving into an essential feature of Schedule Apex in Salesforce . Scheduling tasks in Salesforce can automate repetitive processes, making your life much easier. Whether it’s daily data cleanups or generating weekly reports, Schedulable Apex has got you covered.
Apex is a programming language used in Salesforce. It’s like the brain of Salesforce, telling it what to do and when. With Apex, you can create custom business logic, interact with databases, and automate tasks. It’s powerful and flexible, making it a favorite tool for Salesforce developers.
Imagine manually running the same report every Monday morning or cleaning up data at the end of each day. It can be time-consuming and easy to forget. Scheduling tasks helps automate these repetitive processes, ensuring they run consistently without human intervention. This not only saves time but also reduces the chances of errors.
Table of Contents
Introduction to Schedulable Apex
Schedulable Apex allows you to set up classes to run at specific times. Think of it as setting an alarm clock for your code. You can tell Salesforce to run a piece of code at 2 AM every day or the first of every month. This is incredibly useful for tasks that need to happen regularly or at particular times. For example, if you’re already familiar with Batch Apex, you’ll find Schedulable Apex similarly useful for automating processes.
What is Schedulable Apex?
Schedulable Apex is a feature that lets you schedule the execution of Apex code. You create a special Apex class that implements the Schedulable
interface and then use Salesforce’s scheduling tools to run this class at set times. The main purpose is to automate tasks that need to happen on a schedule.
Common Use Cases for Schedulable Apex
Schedulable Apex is useful in many scenarios. Here are some common ones:
- Daily Data Cleanup: Remove old or unnecessary data from your database to keep it clean and efficient.
- Weekly Report Generation: Automatically create and send reports to your team every week.
- Monthly Billing: Calculate and process billing for customers regularly.
- Data Synchronization: Sync data between Salesforce and other systems at scheduled intervals.
- Maintenance Tasks: Perform regular maintenance, such as recalculating data or updating records.
With Schedulable Apex, these tasks can be automated, freeing up your time for more important activities and ensuring consistency and accuracy. For tasks that require chaining jobs, you might want to explore Queueable Apex.
Scheduling Job for Execution
In this section, we’ll learn two ways to schedule your Apex job for execution: from the Salesforce user interface (UI) and using the System.schedule
method. Let’s get started!
1. Schedule Job from UI
Scheduling a job from the Salesforce UI is straightforward and doesn’t require any coding. Here’s how you can do it:
- Log in to Salesforce, click on the gear icon in the top right corner, and select Setup.
- In the left sidebar, type Apex Classes in the Quick Find box and click on Apex Classes under the Develop section.
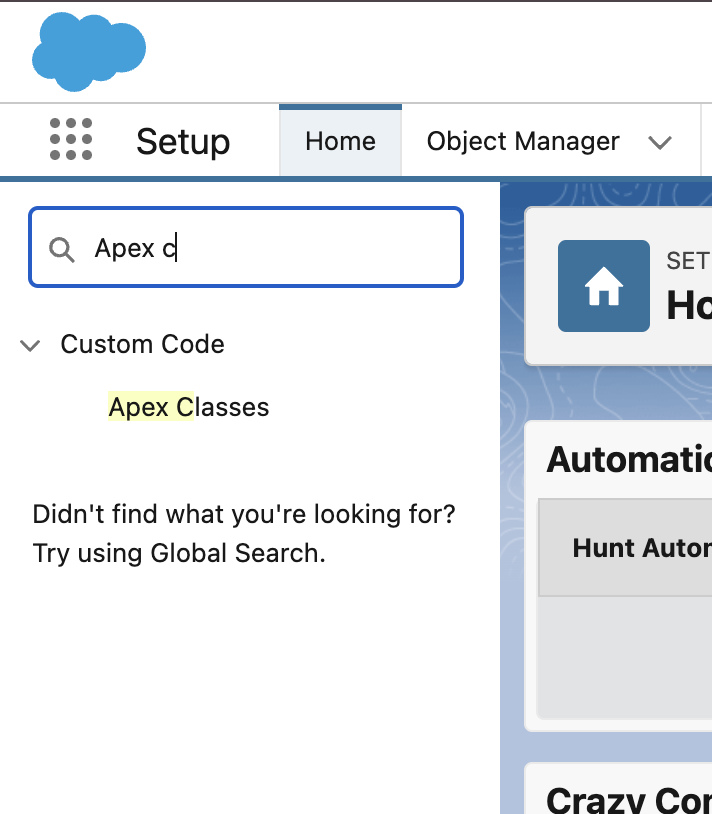
- Click the Schedule Apex button, which you’ll find at the top of the page.
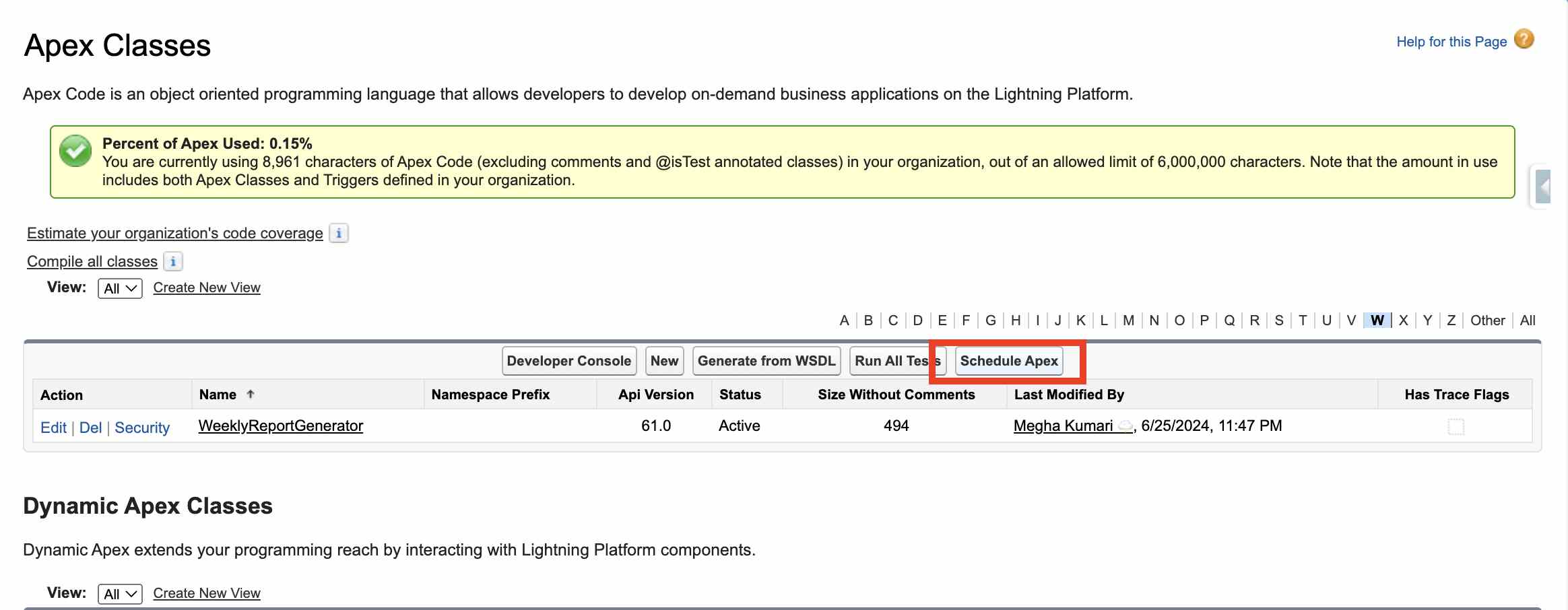
- Fill in the Details:
- Select the Apex class you want to schedule from the dropdown menu. For example, select
WeeklyReportGenerator
. - Enter a name for your Job
- Choose how often the job should run (e.g., daily, weekly).
- Set the start and end dates for your job.
- Choose the time of day when you want the job to run.
- Select the Apex class you want to schedule from the dropdown menu. For example, select
- Click the Save button to schedule the job. Salesforce will now run your Apex class according to the schedule you set.
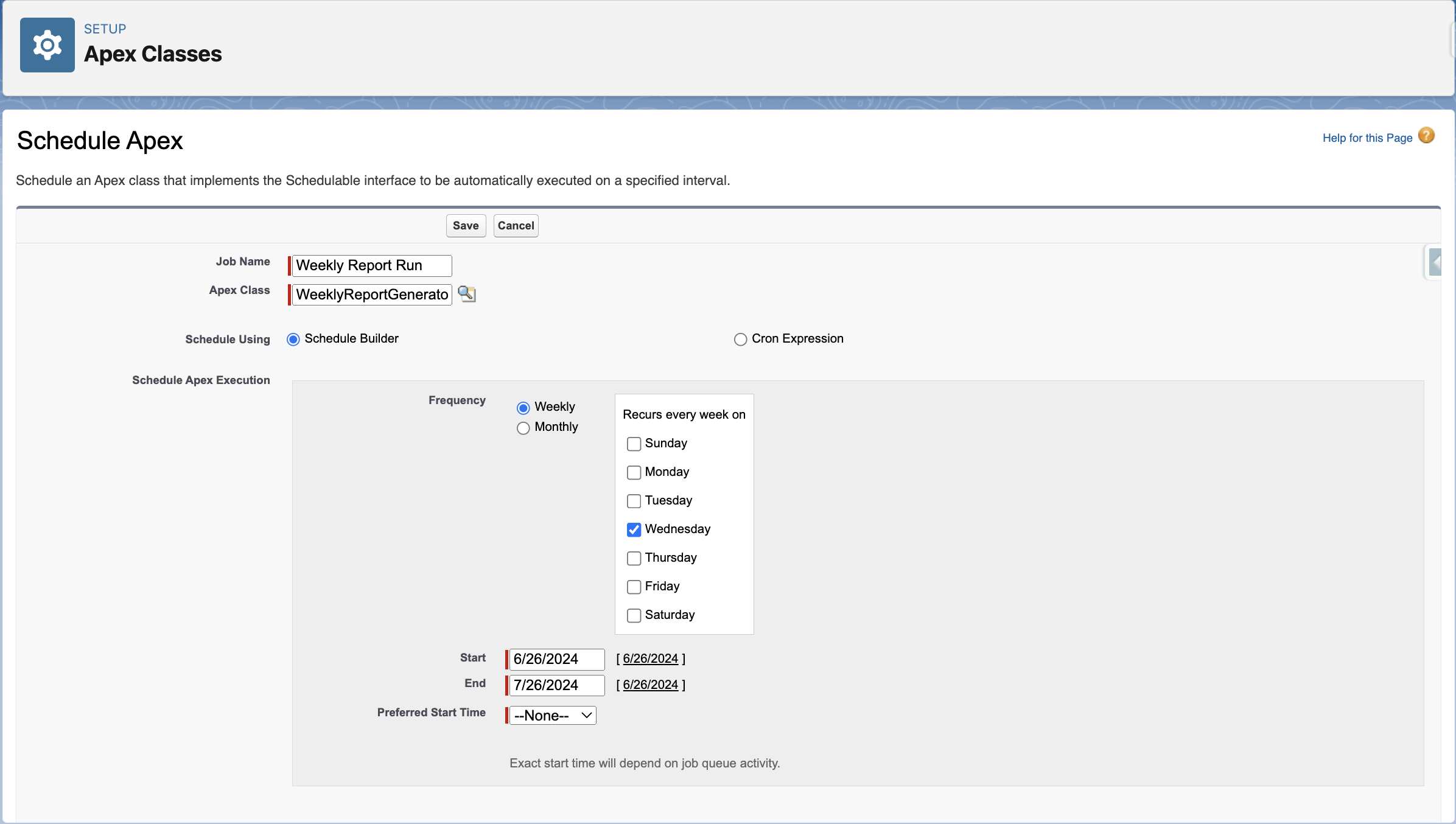
2. Using System.schedule
If you prefer to use code to schedule your job, you can use the System.schedule
method. This method allows you to set up a schedule programmatically using a CRON expression.
Here’s how you can do it:
public class DailyDataCleanup implements Schedulable {
public void execute(SchedulableContext sc) {
// Logic to clean up old data
List<Custom_Object__c> oldRecords = [SELECT Id FROM Custom_Object__c WHERE CreatedDate < LAST_N_DAYS:30];
if (!oldRecords.isEmpty()) {
delete oldRecords;
}
System.debug('Daily data cleanup executed.');
}
}
// Schedule the class to run every day at 2 AM
String cronExpression = '0 0 2 * * ?';
System.schedule('Daily Data Cleanup Job', cronExpression, new DailyDataCleanup());
Detailed Walkthrough:
- The CRON expression
0 0 2 * * ?
tells Salesforce to run the job every day at 2 AM. Here’s a breakdown:0 0 2
means 2:00 AM.*
for day of month means every day.*
for month means every month.?
for day of week means no specific day of the week.
- The
System.schedule
method takes three parameters:- A name for the job, like “Daily Data Cleanup Job.”
- The schedule, like
0 0 2 * * ?
. - An instance of the class you want to run, like
new DailyDataCleanup()
.
String cronExpression = '0 0 2 * * ?'; System.schedule('Daily Data Cleanup Job', cronExpression, new DailyDataCleanup());
By using the System.schedule
method, you can easily schedule your Apex class to run at specific times without needing to use the Salesforce UI.
Monitoring and Managing Scheduled Jobs
After scheduling your Apex classes, it’s important to monitor and manage these scheduled jobs to ensure they run smoothly. You can learn more about managing asynchronous jobs efficiently in our post on Queueable Apex.
Salesforce provides tools to monitor and manage scheduled jobs. Here’s how you can keep an eye on them:
Go to Setup and enter Scheduled Jobs in the Quick Find box. Click on Scheduled Jobs to open the page. Here, you can see all the jobs that are scheduled to run.
- Open Scheduled Jobs
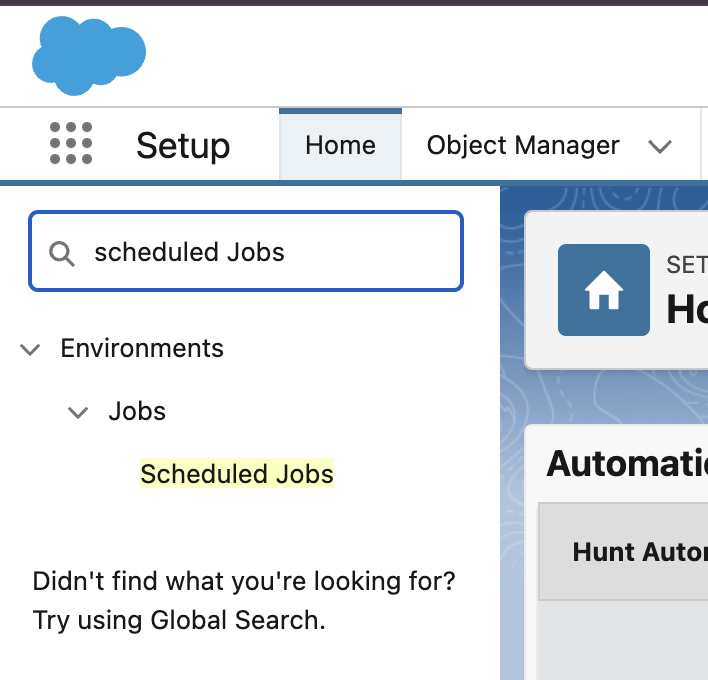
Using the Scheduled Jobs Page
On the Scheduled Jobs page, you can view details about each job, including:
- Job Name
- Job Type
- Next Scheduled Run
- Last Scheduled Run
- Status
You can also manage jobs from this page:
- Pause: Temporarily stop a job from running.
- Resume: Resume a paused job.
- Delete: Remove a job if it’s no longer needed.
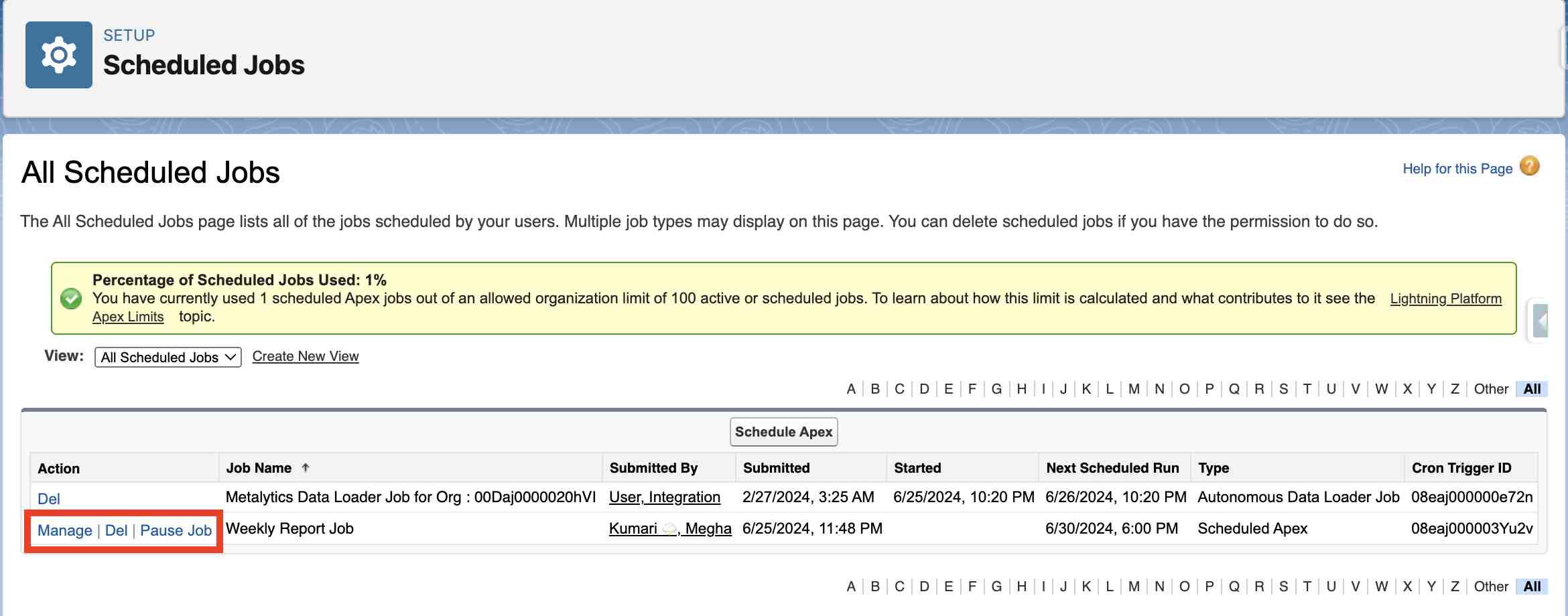
Overview of the Schedulable Interface
The Schedulable interface in Salesforce is like a special set of instructions that tells Salesforce how to run your code at specific times. To use this interface, you need to create a class that implements (or uses) it. This class will contain a method called execute
that Salesforce will run according to the schedule you set.
Step-by-Step Guide to Implementing the Schedulable Interface
- Create an Apex Class:
First, you need to create an Apex class. This class will include the code you want to run on a schedule. - Implement the Schedulable Interface:
To make your class schedulable, you must implement theSchedulable
interface. This involves adding a special line of code to your class. - Write the Execute Method:
Theexecute
method is where you’ll put the code that you want Salesforce to run. This method is required when you implement theSchedulable
interface.
Here’s a simple example to help you understand:
public class MyScheduledClass implements Schedulable {
public void execute(SchedulableContext sc) {
// Your logic here
System.debug('Scheduled Apex executed.');
}
}
Writing a Scheduled Apex Class
Let’s break down the steps to write a scheduled Apex class in detail.
Example Code for a Basic Scheduled Apex Class
public class MyScheduledClass implements Schedulable {
public void execute(SchedulableContext sc) {
// Your logic here
System.debug('Scheduled Apex executed.');
}
}
Explanation of the Code
- Class Declaration:
public class MyScheduledClass implements Schedulable {
This line declares a public class named MyScheduledClass
and tells Salesforce that it implements the Schedulable
interface. By doing this, we make the class schedulable.
- Execute Method:
public void execute(SchedulableContext sc) {
// Your logic here
System.debug('Scheduled Apex executed.');
}
This method is required when implementing the Schedulable
interface. It contains the code that Salesforce will run at the scheduled times. In this example, the System.debug
line is a placeholder for your actual logic. It simply writes a message to the debug log to indicate that the scheduled Apex has run.
Key Components: Execute Method, Logging, and Business Logic
- Execute Method:
This is the main method that runs your code. It takes one parameter,SchedulableContext sc
, which provides context about the scheduled job. - Logging:
UsingSystem.debug
, you can log messages to help you understand what’s happening when your code runs. This is useful for troubleshooting and ensuring that your scheduled tasks are executing as expected. - Business Logic:
The actual work your code needs to do goes here. This could be anything from cleaning up data to generating reports. In the example above, we haven’t included any real business logic, but in your actual class, you would replace theSystem.debug
line with the code that performs the desired task. For more complex scenarios involving triggers, check out our post on Apex Trigger Examples.
Now that we have our basic scheduled Apex class, the next step is to learn how to schedule it to run at specific times. We’ll cover that in the following section.
CRON Expressions
CRON expressions are powerful tools for scheduling. Let’s explore them in more detail.
Detailed Explanation of CRON Expressions
A CRON expression is made up of six fields:
- Seconds (0-59): The second of the minute.
- Minutes (0-59): The minute of the hour.
- Hours (0-23): The hour of the day.
- Day of Month (1-31): The day of the month.
- Month (1-12 or JAN-DEC): The month of the year.
- Day of Week (0-6 or SUN-SAT): The day of the week.
Each part can have specific values or special characters:
*
means “every” (e.g., every minute, every day).?
means “no specific value” (used in day of month and day of week fields).
Common CRON Expression Examples
- Daily at noon:
0 0 12 * * ?
- Every day at 12:00 PM
- Every Monday at 8 AM:
0 0 8 ? * MON
- Every Monday at 8:00 AM
- First day of every month at midnight:
0 0 0 1 * ?
- The first day of every month at 12:00 AM
How to Customize CRON Expressions for Specific Needs
You can customize CRON expressions to fit your specific scheduling needs. Here are a few examples:
- Every hour on the hour:
0 0 * * * ?
- Every hour at the start of the hour (e.g., 1:00, 2:00, etc.)
- Every 15 minutes:
0 0/15 * * * ?
- Every 15 minutes (e.g., 12:00, 12:15, 12:30, etc.)
- Every weekday at 9 AM:
0 0 9 ? * MON-FRI
- Monday to Friday at 9:00 AM
By adjusting the fields in the CRON expression, you can schedule your Apex class to run at almost any time you need.
Real-Life Examples
Let’s look at two real-life examples of how to use Schedulable Apex. These examples will show you how to set up daily data cleanup and weekly report generation tasks.
Example 1: Daily Data Cleanup Task
Imagine you want to clean up old or unnecessary data in your Salesforce database every day. This helps keep your database clean and efficient. Here’s how you can do it:
public class DailyDataCleanup implements Schedulable {
public void execute(SchedulableContext sc) {
// Logic to clean up old data
List<Custom_Object__c> oldRecords = [SELECT Id FROM Custom_Object__c WHERE CreatedDate < LAST_N_DAYS:30];
delete oldRecords;
System.debug('Daily data cleanup executed.');
}
}
// Schedule the class to run every day at 2 AM
String cronExpression = '0 0 2 * * ?';
System.schedule('Daily Data Cleanup Job', cronExpression, new DailyDataCleanup());
Explanation:
- Class Declaration:
public class DailyDataCleanup implements Schedulable {
This line declares a public class named DailyDataCleanup
that implements the Schedulable
interface.
- Execute Method:
public void execute(SchedulableContext sc) {
// Logic to clean up old data
List<Custom_Object__c> oldRecords = [SELECT Id FROM Custom_Object__c WHERE CreatedDate < LAST_N_DAYS:30];
delete oldRecords;
System.debug('Daily data cleanup executed.');
}
In this method, we select records from a custom object that are older than 30 days and delete them. The System.debug
line logs a message to indicate that the cleanup has been executed.
- Scheduling the Class:
String cronExpression = '0 0 2 * * ?';
System.schedule('Daily Data Cleanup Job', cronExpression, new DailyDataCleanup());
This code schedules the DailyDataCleanup
class to run every day at 2 AM using the CRON expression 0 0 2 * * ?
.
Example 2: Weekly Report Generation
Now, let’s say you want to generate a report every week and send it to your team. Here’s how you can do that:
public class WeeklyReportGenerator implements Schedulable {
public void execute(SchedulableContext sc) {
// Logic to generate and send the report
List<Account> accounts = [SELECT Name, AnnualRevenue FROM Account WHERE AnnualRevenue > 1000000];
String report = 'Weekly Report:\n';
for (Account acc : accounts) {
report += 'Account: ' + acc.Name + ', Revenue: ' + acc.AnnualRevenue + '\n';
}
// Pretend to send the report via email
System.debug(report);
System.debug('Weekly report generated and sent.');
}
}
// Schedule the class to run every Sunday at 6 PM
String cronExpression = '0 0 18 ? * SUN';
System.schedule('Weekly Report Job', cronExpression, new WeeklyReportGenerator());
Explanation:
- Class Declaration:
public class WeeklyReportGenerator implements Schedulable {
This line declares a public class named WeeklyReportGenerator
that implements the Schedulable
interface.
- Execute Method:
public void execute(SchedulableContext sc) {
// Logic to generate and send the report
List<Account> accounts = [SELECT Name, AnnualRevenue FROM Account WHERE AnnualRevenue > 1000000];
String report = 'Weekly Report:\n';
for (Account acc : accounts) {
report += 'Account: ' + acc.Name + ', Revenue: ' + acc.AnnualRevenue + '\n';
}
// Pretend to send the report via email
System.debug(report);
System.debug('Weekly report generated and sent.');
}
In this method, we query accounts with annual revenue greater than $1,000,000, generate a report, and log it using System.debug
.
- Scheduling the Class:
String cronExpression = '0 0 18 ? * SUN';
System.schedule('Weekly Report Job', cronExpression, new WeeklyReportGenerator());
This code schedules the WeeklyReportGenerator
class to run every Sunday at 6 PM using the CRON expression 0 0 18 ? * SUN
.
Best Practices for Schedulable Apex
Using Schedulable Apex in Salesforce can make your tasks run smoothly and on time. However, it’s important to follow some best practices to ensure your code is efficient, reliable, and error-free. Let’s explore these best practices in detail.
Writing Efficient and Reliable Code
Efficiency is key when writing Schedulable Apex. Here are some tips to help you write efficient and reliable code:
- Bulkify Your Code:
Make sure your code can handle multiple records at once. This is called “bulkification” For example, if your code processes records, use collections like lists or sets to handle many records in a single run.
public class DailyDataCleanup implements Schedulable {
public void execute(SchedulableContext sc) {
List<Custom_Object__c> oldRecords = [SELECT Id FROM Custom_Object__c WHERE CreatedDate < LAST_N_DAYS:30];
if (!oldRecords.isEmpty()) {
delete oldRecords;
}
System.debug('Daily data cleanup executed.');
}
}
- Optimize Queries:
Use selective queries to fetch only the data you need. Avoid querying unnecessary fields or records.
List<Custom_Object__c> oldRecords = [SELECT Id FROM Custom_Object__c WHERE CreatedDate < LAST_N_DAYS:30 LIMIT 1000];
- Limit DML Operations:
Avoid performing too many DML (Data Manipulation Language) operations in a single transaction. Aim to stay within Salesforce governor limits.
Scheduling Considerations
When scheduling your Apex jobs, consider the following:
- Frequency:
Determine how often your job needs to run. Daily, weekly, or monthly schedules are common, but choose what best fits your needs.
String cronExpression = '0 0 2 * * ?'; // Every day at 2 AM
System.schedule('Daily Data Cleanup Job', cronExpression, new DailyDataCleanup());
- Limits:
Salesforce has limits on the number of scheduled jobs you can have at one time. Be mindful of these limits when scheduling multiple jobs. - Job Timing:
Schedule jobs during off-peak hours to reduce the impact on system performance. For example, running a job late at night might be better than during business hours.
Conclusion
Scheduling Apex in Salesforce is a powerful way to automate tasks and keep your system running smoothly. I encourage you to experiment with scheduling Apex in your org. Try setting up a simple job and see how it works. If you have any questions or feedback, feel free to share them in the comments below or reach out to me directly.
Thanks for reading, and happy scheduling!