Batch Apex in Salesforce is a powerful feature that allows you to process large volumes of data in chunks, making it ideal for long-running operations. Batch Apex is a powerful tool in Salesforce that helps you work with large amounts of data. Imagine you have to process 1 million records of Account data to update a specific field. Doing this in a single transaction is not possible due to governor limits in Salesforce. Instead, you can use Batch Apex to handle this task efficiently. For those just getting started with Salesforce, setting up a free Salesforce developer org can be a great first step. If you are preparing for an interview, our guide on Batch Apex Interview Questions & Answers will help you understand key concepts and practical applications. Additionally, exploring a batch apex interview questions can provide you with practical insights and enhance your understanding of how to implement Batch Apex effectively.
Unlike regular Apex, which has a governor limit on the number of records that can be processed in a single transaction, Batch Apex processes records in smaller chunks (batches), allowing you to work with millions of records efficiently.
Table of Contents
Key Components of Batch Apex
- Batchable Interface: To use Batch Apex, you need to implement the
Database.Batchable
interface in your Apex class. This interface has three methods:start()
: Prepares the batch job, usually by querying the records to be processed.execute()
: Processes each batch of records.finish()
: Finalizes the batch job, often used for post-processing tasks like sending notifications.
For a detailed guide on registering for Salesforce certification exams, you might find this post helpful.
- Batch Size: You can specify the batch size when you run the batch job. The default batch size is 200 records, but you can set it between 1 and 2000 records.
- Governor Limits: Batch Apex runs within specific governor limits for each batch, meaning that each execute method call gets its own set of limits. This helps in avoiding hitting the overall governor limits for your entire job.
Certainly! Here is a list of over 30 interview questions on Batch Apex in Salesforce, along with their answers, to help you prepare thoroughly.
batch apex scenario-based Basic Questions
What is Batch Apex in Salesforce?
Batch Apex is a way to handle large volumes of records in Salesforce by processing them in smaller, manageable chunks. It is useful for long-running operations and bulk data processing, overcoming governor limits that apply to single transactions.
Why would you use Batch Apex instead of a regular Apex class?
Batch Apex is used instead of a regular Apex class when dealing with large datasets that would otherwise exceed governor limits. It allows the processing of millions of records efficiently by dividing them into smaller batches.
How do you implement a Batch Apex class?
To implement a Batch Apex class, you need to implement the Database.Batchable
interface and define three methods: start
, execute
, and finish
. The start
the method prepares the batch job, execute
processes each batch, and finish
handles post-processing tasks.
Explain Start
method in Batch Apex.
The start
method initializes the batch job and returns the scope of records to be processed, typically using Database.getQueryLocator
or a simple list. It is called once at the beginning of the batch job.
Explain the execute
method in Batch Apex.
The execute
method processes each batch of records. It is called repeatedly for each chunk of records and contains the logic to perform the required operations on those records.
Explain the finish
method in Batch Apex.
The finish
method is called after all batches have been processed. It is used for post-processing tasks such as sending confirmation emails or initiating another batch job.
What is the default batch size in Batch Apex?
The default batch size in Batch Apex is 200 records per batch. However, you can specify a different batch size when executing the batch job.
How do you execute a Batch Apex job?
- A Batch Apex job is executed using the
Database.executeBatch
method. You can specify the batch size as a second parameter.
Can Batch Apex be used to delete records?
- Yes, Batch Apex can be used to delete records. In the
execute
method, you can call thedelete
operation on the records passed in the scope.
What Is The Purpose Of The Database.QueryLocator
In Batch Apex?
The Database.QueryLocator
is used in the start
method to efficiently fetch large datasets that need to be processed. It helps in handling large result sets that can be processed in smaller chunks.
public void execute(Database.BatchableContext BC, List<Account> scope)
{
delete scope;
}
How do you execute a Batch Apex job?
- A Batch Apex job is executed using the
Database.executeBatch
method. You can specify the batch size as a second parameter.
UpdateAccountBatch batch = new UpdateAccountBatch();
Database.executeBatch(batch, 100); // Here, batch size is set to 100
batch apex scenario-based Intermediate Questions
How does Batch Apex handle governor limits?
Batch Apex helps manage governor limits by processing records in smaller chunks, each with its own set of governor limits. This prevents hitting limits that apply to single transactions and allows efficient processing of large datasets.
What are some best practices for writing efficient Batch Apex?
Best practices for writing efficient Batch Apex include:
1. Using efficient SOQL queries.
2. Handling exceptions properly.
3. Using smaller batch sizes if necessary.
4. Avoiding complex logic within the execute
method.
5. Ensuring records are processed in a manner that does not exceed governor limits.
How do you test a Batch Apex class?
- Testing a Batch Apex class involves writing test methods that include
Test.startTest
andTest.stopTest
to simulate the execution of the batch job. You should also verify the results to ensure the batch job performs as expected.
@isTest public class UpdateAccountBatchTest {
@isTest static void testBatch() {
// Prepare test data
List<Account> accounts = new List<Account>();
for (Integer i = 0; i < 200; i++) {
accounts.add(new Account(Name = 'Test Account ' + i));
}
insert accounts;
// Execute batch job
UpdateAccountBatch batch = new UpdateAccountBatch();
Test.startTest();
Database.executeBatch(batch, 100);
Test.stopTest();
// Verify results
for (Account acc : [SELECT SomeField__c FROM Account WHERE Id IN :accounts]) {
System.assertEquals('UpdatedValue', acc.SomeField__c);
}
}
}
How do you handle errors in Batch Apex?
Errors in Batch Apex can be handled using try-catch blocks within the execute
method. You can log errors to a custom object or send notifications to administrators. Additionally, you can implement retry logic if needed.
Can you chain Batch Apex jobs? How?
Yes, you can chain Batch Apex jobs by initiating another batch job in the finish
method of the current batch job.
public void finish(Database.BatchableContext BC) {
AnotherBatchJob anotherBatch = new AnotherBatchJob();
Database.executeBatch(anotherBatch);
}
What is the maximum number of batch jobs that can be queued or active simultaneously?
Salesforce allows up to 5 batch jobs to be queued or active simultaneously. If more jobs are submitted, they are held in the Apex flex queue.
What is the Apex flex queue?
The Apex flex queue is a queue that holds batch jobs that are waiting to be processed. It allows you to submit more than 5 batch jobs, and the additional jobs are processed as earlier jobs complete.
How can you monitor the progress of a Batch Apex job?
You can monitor the progress of a Batch Apex job using the Salesforce user interface (Apex Jobs page in Setup) or programmatically by querying the AsyncApexJob
object.
What is the difference between Database.QueryLocator
and Iterable
in the start
method?
Database.QueryLocator
is used for large result sets, allowing efficient handling of millions of records. Iterable
is used for smaller sets of data or when you want to customize the data fetching process.
How do you handle large data volumes in Batch Apex?
Handling large data volumes in Batch Apex involves using Database.QueryLocator
in the start
method, setting appropriate batch sizes, and ensuring efficient SOQL queries. It also includes handling governor limits and optimizing the execute
method.
How do you track the status of the batch class
When we execute the batch we get the job ID by which we can track the status of the batch class. There are two ways by which we can track the status.
1. From UI Apex Job – Navigate to setup –> Quick Find Box –> Search for Apex Job
2. Query on AsyncApexJob
AsyncApexJob aaj = [SELECT Id, Status, JobItemsProcessed, TotalJobItems, NumberOfErrors FROM AsyncApexJob WHERE ID =: batchprocessid ];
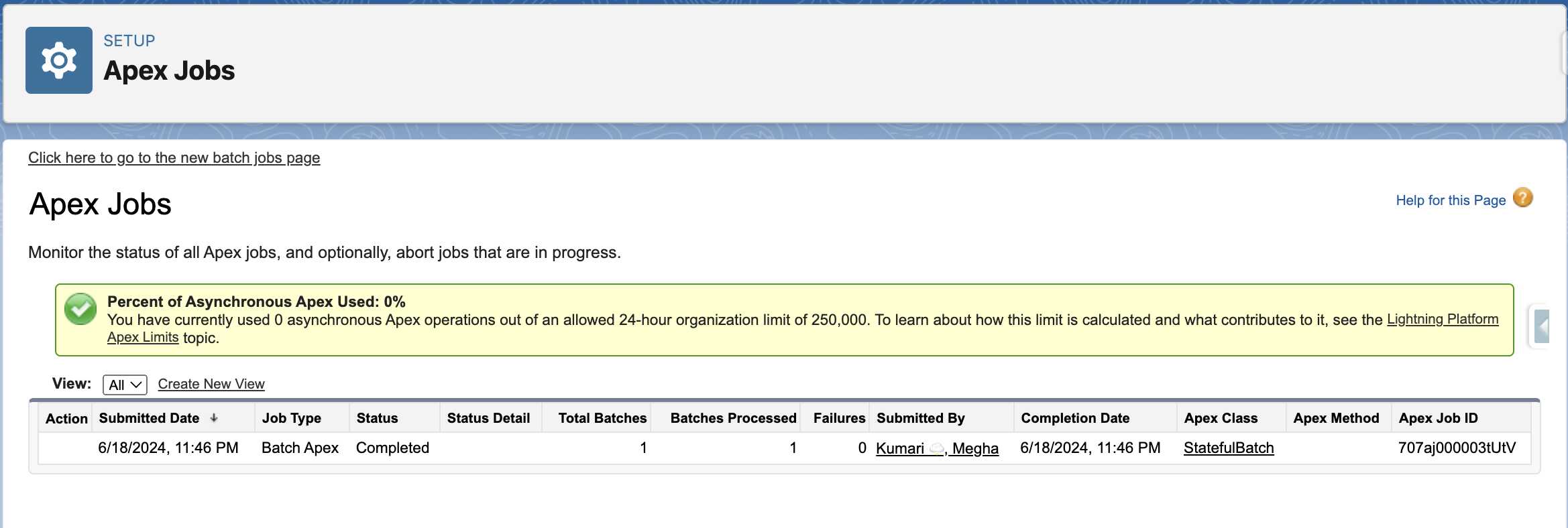
Is it possible to reorder the batch class?
Batch class executes based on “first-in, first-out” in the order they have submitted. If we want to reorder the batch to make it process the first whenever the resources get available, we can do that in two ways:
1. From UI Apex Flex Queue – Batch Jobs that are in holding status go into the flex queue. To navigate to the Apex Flex queue, go to setup –> Quick Find box –> Apex Flex Queue
2. By using System.FlexQueue methods
Boolean isSuccess = System.FlexQueue.moveBeforeJob(jobToMoveId, jobInQueueId);
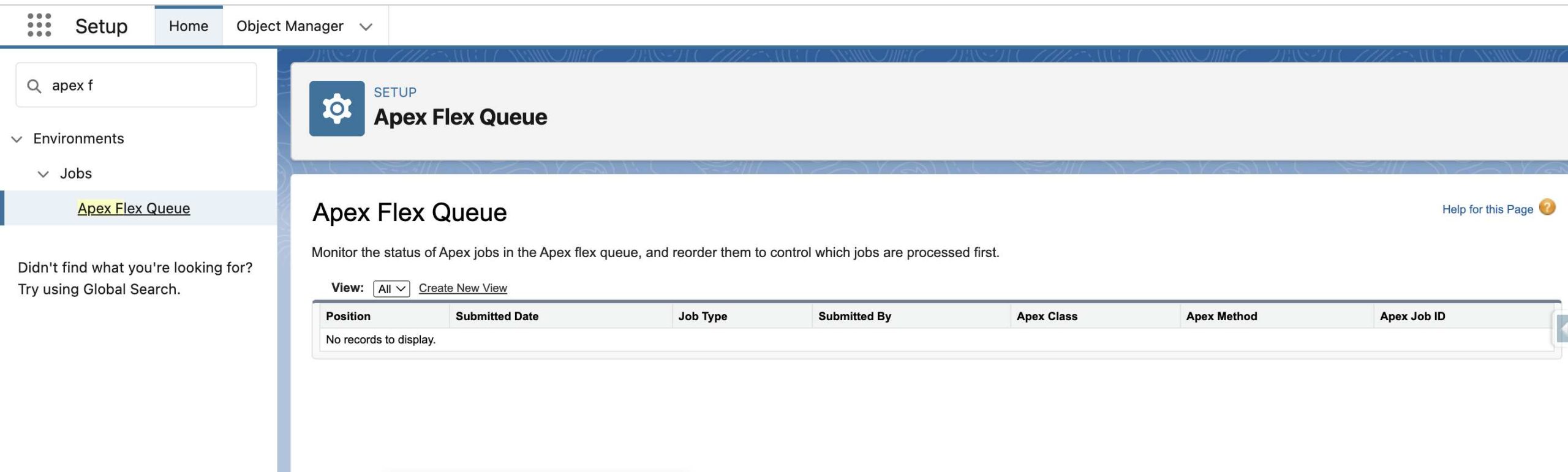
batch apex scenario-based Advanced Questions
Explain a scenario where you would use Batch Apex over other asynchronous processes like Queueable or Future methods.
Batch Apex is preferred when processing large volumes of records that exceed governor limits for synchronous transactions or other asynchronous processes. For example, updating millions of records or performing data clean-up operations across a large dataset. If you’re preparing for an interview that involves complex Salesforce scenarios, you might find these Apex Trigger scenario interview questions helpful.
How do you handle transactions in Batch Apex?
Each batch in Batch Apex runs in its own transaction, ensuring that if a batch fails, only the records in that batch are rolled back. This isolation helps manage large operations without affecting the entire dataset.
Can Batch Apex be scheduled? How?
- Yes, Batch Apex can be scheduled using the
System.scheduleBatch
method or by implementing theSchedulable
interface and callingDatabase.executeBatch
within theexecute
method of theSchedulable
class.
public class ScheduledBatch implements Schedulable {
public void execute(SchedulableContext sc) {
UpdateAccountBatch batch = new UpdateAccountBatch();
Database.executeBatch(batch, 100);
}
}
// To schedule the batch S
cheduledBatch scheduleBatch = new ScheduledBatch();
String cron = '0 0 0 * * ?';
// Every day at midnight
System.schedule('DailyBatchJob', cron, scheduleBatch);
How do you manage batch sizes dynamically?
You can manage batch sizes dynamically by passing the batch size as a parameter when executing the batch job or by adjusting the batch size based on system resources or data volume.
What are some common pitfalls to avoid when using Batch Apex?
Common pitfalls include:
1. Inefficient SOQL queries.
2. Not handling exceptions properly.
3. Using large batch sizes that exceed governor limits.
4. Failing to test batch jobs with realistic data volumes.
Explain the role of Database.Stateful
in Batch Apex.
- Implementing
Database.Stateful
allows you to maintain state across multiple transactions in Batch Apex. It enables you to keep track of stateful information, such as counts or aggregate results, throughout the batch job.
public class StatefulBatch implements Database.Batchable<SObject>, Database.Stateful {
public Integer recordCount = 0; public Database.QueryLocator start(Database.BatchableContext BC) {
return Database.getQueryLocator('SELECT Id FROM Account');
}
public void execute(Database.BatchableContext BC, List<SObject> scope) {
recordCount += scope.size();
}
public void finish(Database.BatchableContext BC) {
System.debug('Total records processed: ' + recordCount);
}
}
How do you ensure data consistency in Batch Apex?
Ensuring data consistency in Batch Apex involves:
1. Using appropriate isolation levels.
2. Implementing proper error handling and retry logic.
3. Ensuring records are processed in a manner that maintains data integrity.
Describe a real-world scenario where you used Batch Apex.
One real-world scenario is performing a data migration project where we had to update millions of Account records with new data from an external system. Batch Apex allowed us to process these records in manageable chunks, ensuring data consistency and compliance with governor limits.
How do you optimize the performance of a Batch Apex job?
Optimizing the performance of a Batch Apex job involves:
1. Writing efficient SOQL queries.
2. Using selective queries with indexed fields.
3. Reducing the batch size if necessary.
4. Avoiding complex logic in the execute
method.
5. Implementing proper indexing and selective data retrieval.
What are the limits for the number of records processed in Batch Apex?
Each transaction in Batch Apex can process up to 50 million records in total. The execute
method can process up to 10,000 records per transaction, but the actual batch size is usually smaller to avoid hitting governor limits.
Can you use Batch Apex for callouts to external systems? How?
- Yes, Batch Apex can be used for callouts to external systems. You need to set the
Database.AllowsCallouts
interface on the Batch Apex class and handle callouts within theexecute
method. Remember to manage callout limits and governor limits.
public class CalloutBatch implements Database.Batchable<SObject>, Database.AllowsCallouts {
public Database.QueryLocator start(Database.BatchableContext BC) {
return Database.getQueryLocator('SELECT Id FROM Account');
}
public void execute(Database.BatchableContext BC, List<SObject> scope) {
for (SObject sObj : scope) {
// Perform callout
HttpRequest req = new HttpRequest();
req.setEndpoint('https://api.example.com/data');
req.setMethod('GET'); Http http = new Http(); ]
HttpResponse res = http.send(req); // Process response
}
}
public void finish(Database.BatchableContext BC) {
System.debug('Batch job with callouts complete.');
}
}
What are some considerations when using Batch Apex in a multi-tenant environment?
In a multi-tenant environment, consider the impact on shared resources. Optimize your Batch Apex jobs to be efficient and avoid excessive resource usage. Also, respect governor limits and design jobs to be scalable and considerate of other users on the platform.
How do you handle long-running Batch Apex jobs?
Long-running Batch Apex jobs can be managed by:
1. Breaking the job into smaller, manageable batches.
2. Using job chaining to continue processing after one batch job is completed.
3. Monitoring and optimizing performance to ensure the job is completed within reasonable timeframes.
What is the impact of running multiple Batch Apex jobs concurrently?
Running multiple Batch Apex jobs concurrently can lead to increased resource usage and potential contention. It’s important to monitor resource usage and prioritize jobs effectively using the flex queue to avoid performance degradation.
How can you improve the reliability of Batch Apex jobs?
Improving the reliability of Batch Apex jobs involves:
1. Implementing robust error handling and logging.
2. Using retry mechanisms for transient errors.
3. Monitoring job performance and adjusting batch sizes as needed.
4. Ensuring test coverage and realistic test scenarios.
Conclusion
Mastering Batch Apex is essential for any Salesforce developer, especially when dealing with large volumes of data and complex business processes. Understanding how to implement, optimize, and manage Batch Apex jobs not only helps in overcoming Salesforce governor limits but also ensures efficient and scalable solutions.
In this guide, we’ve covered a comprehensive set of interview questions and answers, ranging from basic concepts like the implementation of the Database.Batchable
interface to advanced topics such as error handling, chaining batch jobs, and making callouts in Batch Apex. By familiarizing yourself with these questions, you can confidently demonstrate your knowledge and expertise during interviews.
Remember, the key to excelling in Batch Apex interviews is not just knowing the theoretical aspects but also being able to provide real-world examples and best practices. Highlighting your experience with practical scenarios and explaining how you’ve tackled complex problems will set you apart from other candidates. For further reading, you might want to check out my post on LWC interview questions.
As you prepare for your interview, focus on understanding the underlying principles of Batch Apex, practicing with different scenarios, and optimizing your solutions to handle large data volumes efficiently. With thorough preparation and a solid grasp of these concepts, you’ll be well-equipped to impress your interviewers and secure your next Salesforce development role.
Good luck with your interview preparation, and may you continue to build innovative and robust solutions in the Salesforce ecosystem!