Have you ever wished you could automatically sum up data from related records in Salesforce? For example, imagine you have an Invoice
object and an Invoice Line Item
object. Wouldn’t it be great if the total amount from all Invoice Line Items
could automatically show up on the Invoice
? That’s where roll-up summary fields come in handy.
But there’s a catch: roll-up summary fields only work for master-detail relationships, not lookup relationships. So, what do you do if you’re using a lookup relationship? Don’t worry, I’ve got you covered!
Roll-up summary fields in Salesforce are powerful tools for calculating values from related records. They can sum up numbers, count records, find the minimum or maximum value, and more. However, they only work when the relationship between objects is master-detail. This limitation can be a headache if you’re working with lookup relationships, which are more flexible and often used in real-world scenarios.
Table of Contents
What is Roll-up Summary Fields?
Roll-up summary fields in Salesforce allow you to perform calculations on related records and display the result on a parent record. For example, if you have an Account
with multiple Opportunities
, a roll-up summary field on the Account
can show the total value of all Opportunities
.
Roll-up summary fields are commonly used in various scenarios, such as:
- Summing up the total value of
Opportunities
for anAccount
. - Counting the number of
Contacts
related to anAccount
. - Finding the highest or lowest
Amount
in relatedOpportunity
records.
Here’s a screenshot showing a roll-up summary field in action. In this example, we have an Account
with multiple Opportunities
. The Total Opportunity Amount
field on the Account
is a roll-up summary field that sums up the Amount
of all related Opportunities
.
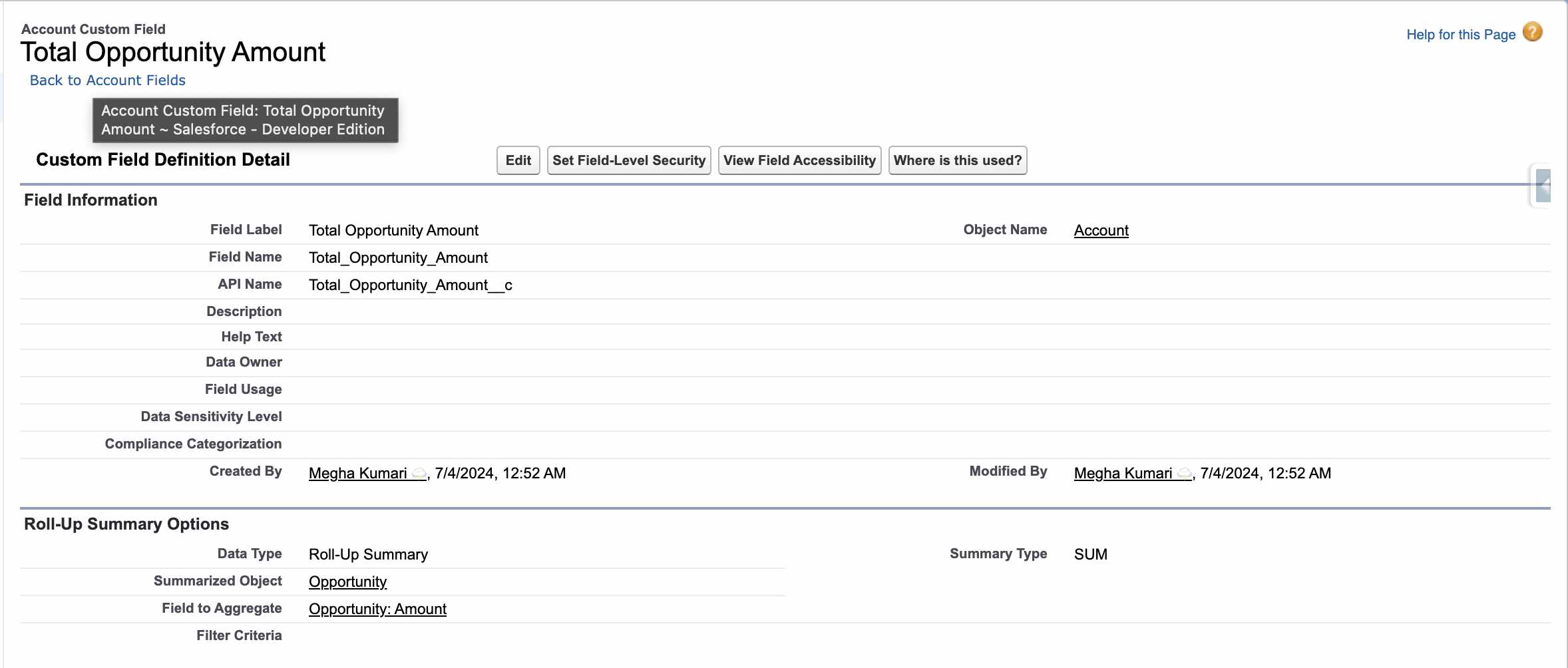
This image demonstrates how a roll-up summary field can display the total amount from related records. Unfortunately, this functionality is limited to master-detail relationships, and we’ll need to create a custom trigger for lookup relationships.
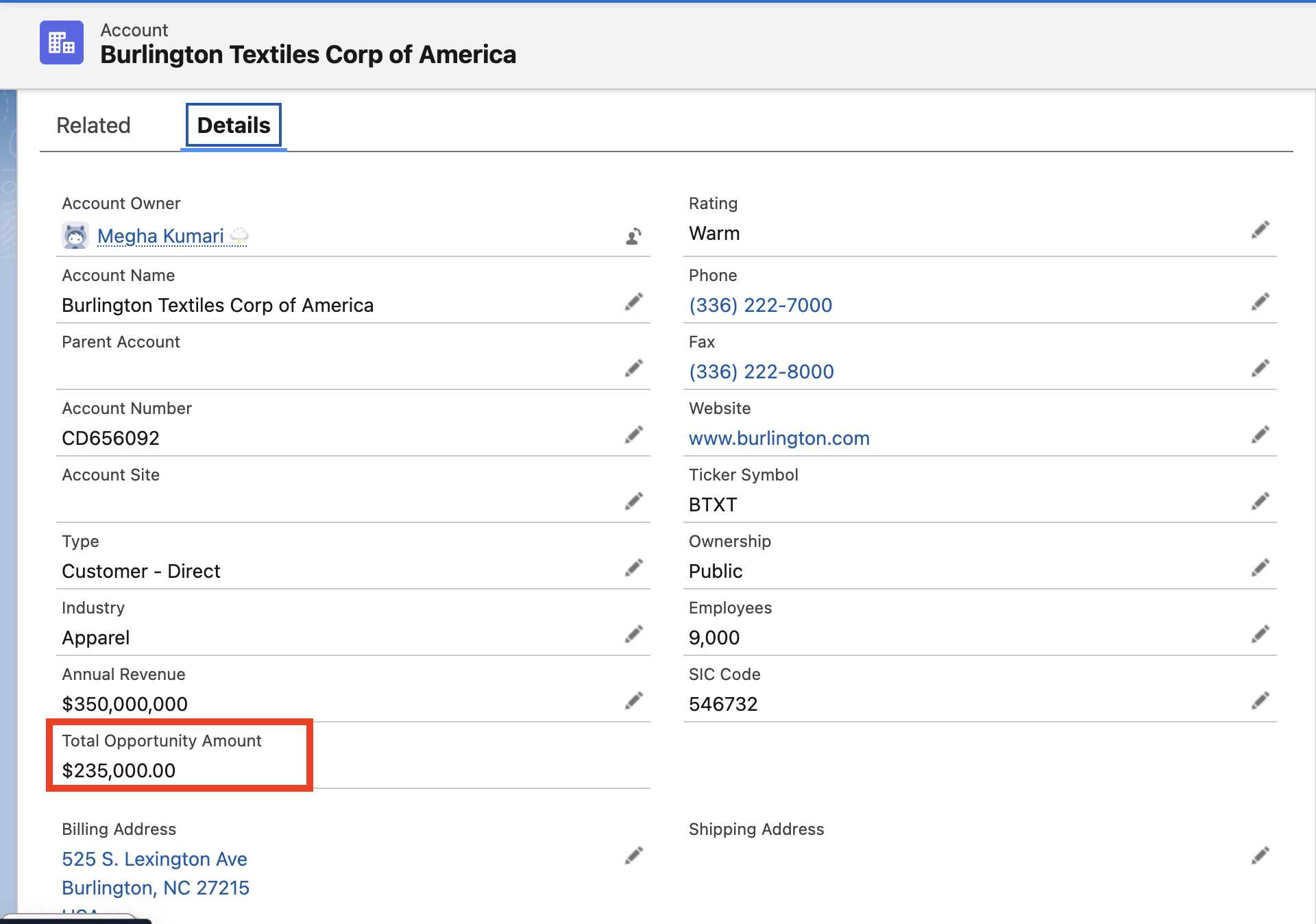
Creating a Roll-up Summary for Lookup Relationships
In the next section, we’ll dive into the steps to create a custom roll-up summary trigger for a lookup relationship. We’ll use a real-world example involving Invoices
and Invoice Line Items
to illustrate the process. Get ready to enhance your Salesforce skills with this powerful custom solution!
Okay, let’s dig into why we need custom roll-up summary triggers for lookup relationships. Imagine you have a bunch of records in Salesforce that are related to each other, but not in a master-detail way. In simpler terms, these records are connected, but one isn’t the boss of the other. This is called a lookup relationship.
In a master-detail relationship, you can easily create a roll-up summary field to calculate totals, counts, averages, etc., from the child records and display them on the parent record. However, Salesforce doesn’t let you do this for lookup relationships. This means if you want to sum up or count data from related records in a lookup relationship, you can’t use the built-in roll-up summary fields. That’s a bummer, right?
But don’t worry, we can create a custom roll-up summary trigger to solve this problem! A trigger is a piece of code that runs automatically when certain events happen in Salesforce, like when records are created, updated, or deleted. By using a trigger, we can manually calculate the roll-up summary and update the parent record in a lookup relationship.
Example Scenario
Let’s walk through a real-world example to understand this better. Imagine you work at a company that handles invoices and invoice line items. Here’s the scenario:
- You have an
Invoice
object that represents a customer’s bill. - You have an
Invoice Line Item
object that represents each item on that bill. - Each
Invoice Line Item
is linked to anInvoice
through a lookup relationship.
Now, you want to see the total amount of all Invoice Line Items
on the Invoice
record. Since this is a lookup relationship, you can’t use the standard roll-up summary field. So, we’ll create a custom roll-up summary trigger to get the job done.
Invoice and Invoice Line Items Relationship
Here’s a simple diagram to show the relationship between Invoice
and Invoice Line Item
:
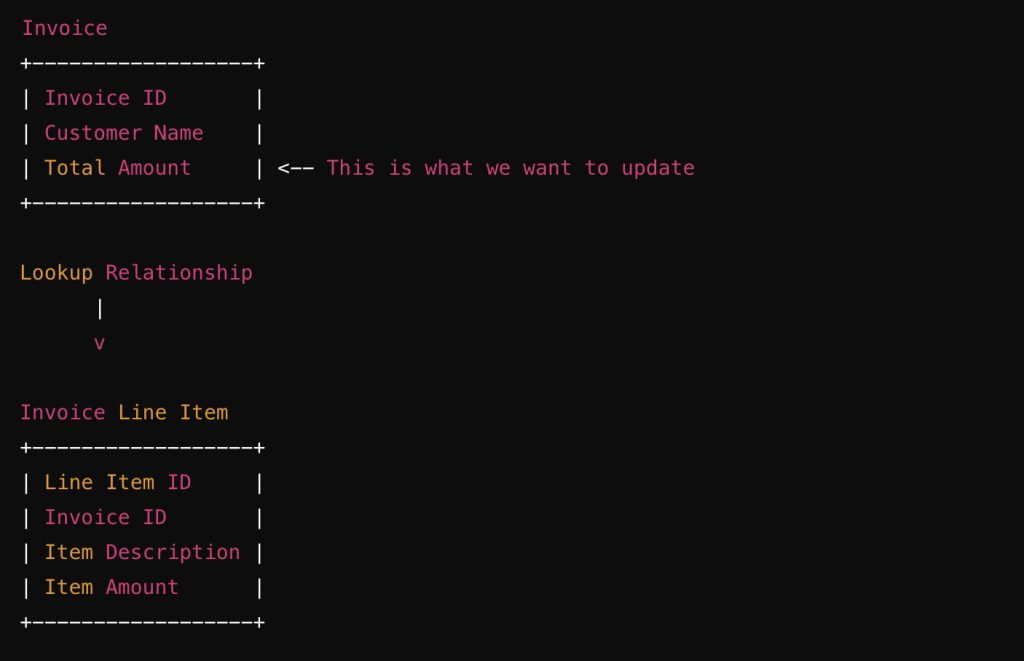
In this example, each Invoice Line Item
is linked to an Invoice
through the Invoice ID
field. We want to sum up the Item Amount
of all Invoice Line Items
and show it in the Total Amount
field on the Invoice
.
Custom Roll-up Summary Trigger
Now, let’s write the trigger to achieve this. We’ll write a trigger on the Invoice Line Item
object that fires whenever an Invoice Line Item
is inserted, updated, or deleted. This trigger will calculate the total amount and update the corresponding Invoice
.
Here is the code snippet for our trigger:
trigger InvoiceLineItemTrigger on Invoice_Line_Item__c (after insert, after update, after delete, after undelete) {
// Set to store Invoice IDs to update
Set<Id> invoiceIds = new Set<Id>();
// Collect Invoice IDs from trigger context
if (Trigger.isInsert || Trigger.isUpdate || Trigger.isUndelete) {
for (Invoice_Line_Item__c item : Trigger.new) {
invoiceIds.add(item.Invoice__c);
}
}
if (Trigger.isDelete) {
for (Invoice_Line_Item__c item : Trigger.old) {
invoiceIds.add(item.Invoice__c);
}
}
// Fetch Invoice records
List<Invoice__c> invoices = [SELECT Id, Total_Invoice_Amount__c FROM Invoice__c WHERE Id IN :invoiceIds];
// Map to store total amounts
Map<Id, Decimal> invoiceTotals = new Map<Id, Decimal>();
// Initialize totals
for (Invoice__c inv : invoices) {
invoiceTotals.put(inv.Id, 0);
}
// Calculate totals
for (Invoice_Line_Item__c item : [SELECT Invoice__c, Amount__c FROM Invoice_Line_Item__c WHERE Invoice__c IN :invoiceIds]) {
if (invoiceTotals.containsKey(item.Invoice__c)) {
invoiceTotals.put(item.Invoice__c, invoiceTotals.get(item.Invoice__c) + item.Amount__c);
}
}
// Update Invoice records
List<Invoice__c> invoicesToUpdate = new List<Invoice__c>();
for (Invoice__c inv : invoices) {
inv.Total_Invoice_Amount__c = invoiceTotals.get(inv.Id);
invoicesToUpdate.add(inv);
}
update invoicesToUpdate;
}
This trigger will ensure that every time an Invoice Line Item
is created, updated, or deleted, the total amount on the corresponding Invoice
is updated accordingly.
Creating a Roll-up Summary Field
We’ll go step-by-step to ensure everything is clear and easy to follow.
First, we need to know which objects we’re working with. In our example, we have:
- Parent Object:
Invoice
- Child Object:
Invoice Line Item
The Invoice
object will hold the total amount, and the Invoice Line Item
object will have individual amounts for each line item.
Next, let’s identify the fields we need:
- On the Parent Object (Invoice):
Total_Invoice_Amount__c
: A custom field to store the total amount of all related invoice line items.
- On the Child Object (Invoice Line Item):
Invoice__c
: A lookup field to the parentInvoice
.Amount__c
: The amount for each line item.
Limitations
While roll-up summary fields are incredibly useful, they come with a significant limitation: they only work for master-detail relationships. In a master-detail relationship, the child record is tightly linked to the parent, who controls the child’s lifecycle. However, in a lookup relationship, the link is looser, and the child can exist independently of the parent. This flexibility is why lookup relationships are often preferred, but it also means we can’t use standard roll-up summary fields.
Conclusion
We started by understanding the importance of roll-up summary fields and their limitation in Salesforce, especially with lookup relationships. Then, we explored why custom roll-up summary triggers are needed and walked through a real-world example with Invoices
and Invoice Line Items
.
We prepared by identifying the objects and fields needed. Next, we created a detailed roll-up summary trigger, handling various events like insert, update, delete, and undelete. We also broke down the trigger code step-by-step to make it easy to understand.
If you enjoyed this post and want to learn more about related topics, check out these helpful resources:
- Salesforce Roll-Up Summary Fields Overview
- Batch Apex Interview Questions
- Verify Your Salesforce Certification
These resources will deepen your understanding and give you more tools to work with in Salesforce.